Salve stavo sviluppando un progetto sulla gestione di thread real time e volevo includervi due librerie in una della quali volevo dichiarare una struttura che userò sia nella definizione delle funzioni di libreria sia all'interno del main. Soltanto mi da problemi in fase di compilazione nel main quando uso questa struttura.
Ecco i file riguardanti la prima libreria:
I file riguardanti la seconda:
Ed ecco il file dove è presente main di prova:
Questo è quello che mi viene scritto nella shell:
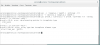
Che significa? Dove ho sbagliato con la struttura e la libreria? :crying:
Ecco i file riguardanti la prima libreria:
Codice:
/*
* taskRT.h
*
* Created on: 04 gen 2016
* Author: veronica
*/
#ifndef TASKRT_H_
typedef struct task_par tp;
#define TASKRT_H_
void set_period(struct task_par *tp);
void wait_for_period(struct task_par *tp);
int deadline_miss(struct task_par *tp);
#endif /* TASKRT_H_ */
Codice:
/*
* taskRT.c
*
* Created on: 04 gen 2016
* Author: veronica
*/
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <time.h>
#include "timeplus.h"
#include "taskRT.h"
struct task_par {
int arg;
long wcet;
int period;
int deadline;
int priority;
int dmiss;
struct timespec at;
struct timespec dl;
};
void set_period(struct task_par *tp)
{
struct timespec t;
clock_gettime(CLOCK_MONOTONIC, &t);
time_copy(&(tp->at), t);
time_copy(&(tp->dl), t);
time_add_ms(&(tp->at), tp->period);
time_add_ms(&(tp->dl), tp->deadline);
}
void wait_for_period(struct task_par *tp)
{
clock_nanosleep(CLOCK_MONOTONIC, TIMER_ABSTIME, &(tp->at), NULL);
time_add_ms(&(tp->at), tp->period);
time_add_ms(&(tp->dl), tp->period);
}
int deadline_miss(struct task_par *tp)
{
struct timespec now;
clock_gettime(CLOCK_MONOTONIC, &now);
if(time_cmp(now, tp->dl) > 0) {
tp->dmiss++;
return 1;
}
return 0;
}
I file riguardanti la seconda:
Codice:
/*
* timeplus.h
*
* Created on: 04 gen 2016
* Author: veronica
*/
#ifndef TIMEPLUS_H_
#define TIMEPLUS_H_
void time_copy(struct timespec *td, struct timespec ts);
void time_add_ms(struct timespec *t, int ms);
int time_cmp(struct timespec t1, struct timespec t2);
#endif /* TIMEPLUS_H_ */
Codice:
* timeplus.c
*
* Created on: 04 gen 2016
* Author: veronica
*/
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <time.h>
#include "timeplus.h"
void time_copy(struct timespec *td, struct timespec ts)
{
td->tv_sec = ts.tv_sec;
td->tv_nsec = ts.tv_nsec;
}
void time_add_ms(struct timespec *t, int ms)
{
t->tv_sec += ms/1000;
t->tv_nsec += (ms%1000)*1000000;
if(t->tv_nsec > 1000000000) {
t->tv_nsec -= 1000000000;
t->tv_sec += 1;
}
}
int time_cmp(struct timespec t1, struct timespec t2)
{
if(t1.tv_sec > t2.tv_sec)
return 1;
if(t1.tv_sec < t2.tv_sec)
return -1;
if(t1.tv_nsec > t2.tv_nsec)
return 1;
if(t1.tv_nsec < t2.tv_nsec)
return -1;
return 0;
}
Ed ecco il file dove è presente main di prova:
Codice:
/*
* proval.c
*
* Created on: 04 gen 2016
* Author: veronica
*/
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <time.h>
#include <allegro.h>
#include "taskRT.h"
#include "timeplus.h"
const int NT=4;
int x,y;
pthread_mutex_t muxsem= PTHREAD_MUTEX_INITIALIZER;
void *task(void *arg)
{
struct task_par *tp;
int i, n, col;
char s[50];
// local state variable
pthread_t tid;
tid = pthread_self();
tp= (struct task_par *)arg;
i=tp->arg;
set_period(tp);
while(1) {
//thread body
sprintf(s,"I am in the thread n: %d tid: %lu",i,tid);
do {
n = rand();
col = n % 16;
}while(col==7);
//fflush(stdout);
pthread_mutex_lock(&muxsem);
y=460-((i*10)+10);
line(screen, x, y, (x+10), y, col);
x=x+10;
if(x==610) {
rectfill(screen, 21, 455, 610, 310, 7);
x=20;
}
textout_ex(screen,font,s,20,20,col,-1);
line(screen, 0, 40, 639, 40, col);
sleep(2);
textout_ex(screen,font,s,20 ,20,7,-1);
pthread_mutex_unlock(&muxsem);
if(deadline_miss(tp))
printf("deadline miss!");
wait_for_period(tp);
}
}
int main (void)
{
struct sched_param mypar;
struct task_par tp[NT];
pthread_attr_t att[NT];
pthread_t tid[NT];
int i, err = 0;
//allegro
allegro_init();
install_keyboard();
set_color_depth(8);
set_gfx_mode(GFX_AUTODETECT_WINDOWED, 640,480,0,0);
clear_to_color(screen, 7);
line(screen, 20, 300, 20, 460, 0);
line(screen, 620, 460, 20, 460, 0);
line(screen, 10, 310, 20, 300, 0);
line(screen, 30, 310, 20, 300, 0);
line(screen, 610, 450, 620, 460, 0);
line(screen, 610, 470, 620, 460, 0);
srand(time(0));
//readkey();
x=20;
for(i=0; i<NT; i++) {
tp[i].arg = i;
tp[i].period = 100;
tp[i].deadline = 80;
tp[i].priority = 20;
tp[i].dmiss = 0;
pthread_attr_init(&att[i]);
err=pthread_attr_setinheritsched(&att[i], PTHREAD_EXPLICIT_SCHED);
if(err > 0) {
perror("pthread_attr_setinheritsched problem\n");
exit(1);
}
err=pthread_attr_setschedpolicy(&att[i], SCHED_FIFO);
if(err > 0) {
perror("pthread_attr_setschedpolicy problem\n");
exit(1);
}
mypar.__sched_priority = tp[i].priority;
err=pthread_attr_setschedparam(&att[i], &mypar);
if(err > 0) {
perror("pthread_attr_setschedparam problem\n");
exit(1);
}
err=pthread_create(&tid[i], &att[i], task, &tp[i]);
if(err > 0) {
perror("pthread create problem\n");
exit(1);
}
}
for(i=0; i<NT; i++) {
err=pthread_join(tid[i], NULL);
if(err > 0)
perror("join problem");
exit(1);
}
for(i=0; i<NT; i++)
pthread_attr_destroy(&att[i]);
allegro_exit();
return 0;
}
Questo è quello che mi viene scritto nella shell:
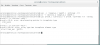
Che significa? Dove ho sbagliato con la struttura e la libreria? :crying: